Introduction
In this tutorial, we will create a simple app to handle incoming WhatsApp messages using python.
Dependencies
install ngrok
for local development purposes, a tunneling service is required. This example uses ngrok , You can download ngrok from here.
install FLASK
pip install flask
we need to deploy the server using the FLASK framework.
The FLASK allows to conveniently respond to incoming requests and process them.
step 1 : Create new flask app
We will create the file: app.py And we write the following code inside it
from flask import Flask, request, jsonify
from test import Ultrawebhook
import json
app = Flask(__name__)
@app.route('/', methods=['POST'])
def home():
if request.method == 'POST':
bot = Ultrawebhook(request.json)
return bot.processing()
if(__name__) == '__main__':
app.run()
step 2 : Incoming message processing
We will create the file: test.py And we write the following code inside it
import json
import requests
class Ultrawebhook():
def __init__(self, json):
self.json = json
self.dict_messages = json['data']
def processing(self):
if self.dict_messages != []:
message = self.dict_messages
msg_from = message['from'].split()
msg_text = message['body'].split()
print("sender phone number : " + msg_from[0])
print("message : " + msg_text[0])
return ''
step 3 : Run the project
Start flask :
flask run
Start ngrok
For Windows :
ngrok http 80
For Mac :
./ngrok http 5000
After this, you should see a for example :
https://7647-115-83-121-164.ngrok.io
paste your URL in Instance settings ، As the following picture
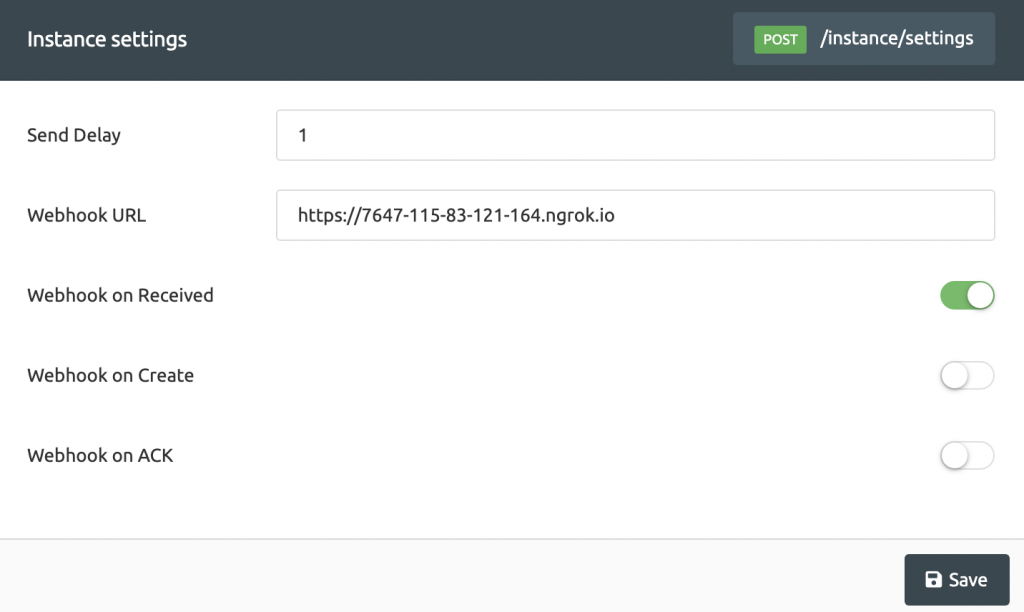
EXAMPLE JSON RESPONSE
{
"event_type": "message_received",
"instanceId": "90",
"data": {
"id": "[email protected]_7ECAED9EB68D3474BE591443134C2E3F",
"from": "[email protected]",
"to": "[email protected]",
"ack": "pending",
"type": "chat",
"body": "I can't send a message using php code\nCan you help me",
"fromMe": false,
"isForwarded": false,
"time": 1643311467
}
}
Finley : Project Test and Receive WhatsApp messages the first message
Now send a WhatsApp message to the number you are linking the instance to.
The sender’s number and the sent message will be printed.
Congratulations. You have received your first WhatsApp message.
Now after receiving WhatsApp messages you can build a chatbot easily using Python and Ultramsg API.
Types of webhooks to use with WhatsApp API & Ultramsg
- webhook_message_received : notifications in webhooks when message received .
- webhook_message_create : notifications in webhooks when message create .
- webhook_message_ack : ack (message delivered and message viewed) notifications in webhooks.
you can see Full Whatsapp API Documentation and FAQ.