creare chatbot è molto semplice con l’API Ultramsg, puoi creare un chatbot del servizio clienti e il miglior chatbot ai attraverso semplici passaggi utilizzando il linguaggio Python.
Chatbot Opportunità e attività del bot WhatsApp
- L’output dell’elenco dei comandi.
- L’output dell’ora del server del bot in esecuzione su .
- Invio dell’immagine al numero di telefono o al gruppo.
- Invio file audio.
- Invio registrazione audio ppt.
- Invio di file video.
- Invio contatto.
Passaggio 1: installare il pallone
dobbiamo distribuire il server usando il framework FLSK.
Il FLASK consente di rispondere comodamente alle richieste in arrivo ed elaborarle.
pip install flask
Passaggio 2: installa ngrok
per scopi di sviluppo locale è necessario un servizio di tunneling. Questo esempio usa ngrok , puoi scaricare ngrok da qui .
Passaggio 3: crea una nuova app per flask
Creeremo il file: app.py e al suo interno scriviamo il seguente codice
from flask import Flask, request, jsonify
from ultrabot import ultraChatBot
import json
app = Flask(__name__)
@app.route('/', methods=['POST'])
def home():
if request.method == 'POST':
bot = ultraChatBot(request.json)
return bot.Processingـincomingـmessages()
if(__name__) == '__main__':
app.run()
Passaggio 4: elaborazione dei messaggi in arrivo
Creeremo il file: ultrabot.py e al suo interno scriviamo il seguente codice
import json
import requests
import datetime
class ultraChatBot():
def __init__(self, json):
self.json = json
self.dict_messages = json['data']
self.ultraAPIUrl = 'https://api.ultramsg.com/{{instance_id}}/'
self.token = '{{token}}'
def send_requests(self, type, data):
url = f"{self.ultraAPIUrl}{type}?token={self.token}"
headers = {'Content-type': 'application/json'}
answer = requests.post(url, data=json.dumps(data), headers=headers)
return answer.json()
def send_message(self, chatID, text):
data = {"to" : chatID,
"body" : text}
answer = self.send_requests('messages/chat', data)
return answer
def send_image(self, chatID):
data = {"to" : chatID,
"image" : "https://file-example.s3-accelerate.amazonaws.com/images/test.jpeg"}
answer = self.send_requests('messages/image', data)
return answer
def send_video(self, chatID):
data = {"to" : chatID,
"video" : "https://file-example.s3-accelerate.amazonaws.com/video/test.mp4"}
answer = self.send_requests('messages/video', data)
return answer
def send_audio(self, chatID):
data = {"to" : chatID,
"audio" : "https://file-example.s3-accelerate.amazonaws.com/audio/2.mp3"}
answer = self.send_requests('messages/audio', data)
return answer
def send_voice(self, chatID):
data = {"to" : chatID,
"audio" : "https://file-example.s3-accelerate.amazonaws.com/voice/oog_example.ogg"}
answer = self.send_requests('messages/voice', data)
return answer
def send_contact(self, chatID):
data = {"to" : chatID,
"contact" : "[email protected]"}
answer = self.send_requests('messages/contact', data)
return answer
def time(self, chatID):
t = datetime.datetime.now()
time = t.strftime('%Y-%m-%d %H:%M:%S')
return self.send_message(chatID, time)
def welcome(self,chatID, noWelcome = False):
welcome_string = ''
if (noWelcome == False):
welcome_string = "Hi , welcome to WhatsApp chatbot using Python\n"
else:
welcome_string = """wrong command
Please type one of these commands:
*hi* : Saluting
*time* : show server time
*image* : I will send you a picture
*video* : I will send you a Video
*audio* : I will send you a audio file
*voice* : I will send you a ppt audio recording
*contact* : I will send you a contact
"""
return self.send_message(chatID, welcome_string)
def Processingـincomingـmessages(self):
if self.dict_messages != []:
message =self.dict_messages
text = message['body'].split()
if not message['fromMe']:
chatID = message['from']
if text[0].lower() == 'hi':
return self.welcome(chatID)
elif text[0].lower() == 'time':
return self.time(chatID)
elif text[0].lower() == 'image':
return self.send_image(chatID)
elif text[0].lower() == 'video':
return self.send_video(chatID)
elif text[0].lower() == 'audio':
return self.send_audio(chatID)
elif text[0].lower() == 'voice':
return self.send_voice(chatID)
elif text[0].lower() == 'contact':
return self.send_contact(chatID)
else:
return self.welcome(chatID, True)
else: return 'NoCommand'
Passaggio 5: avvia il progetto WhatsApp Chatbot
Esegui il server FLSK
flask run
Corri Ngrok
Esegui ngrok per Windows:
ngrok http 5000
Esegui ngrok per Mac:
./ngrok http 5000
Passaggio 6: imposta l’URL Webhook nelle impostazioni dell’istanza
Dopo aver eseguito ngrok, dovresti vedere ad esempio:
https://7647-115-83-121-164.ngrok.io
incolla l’URL nelle impostazioni dell’istanza , Come nell’immagine seguente :
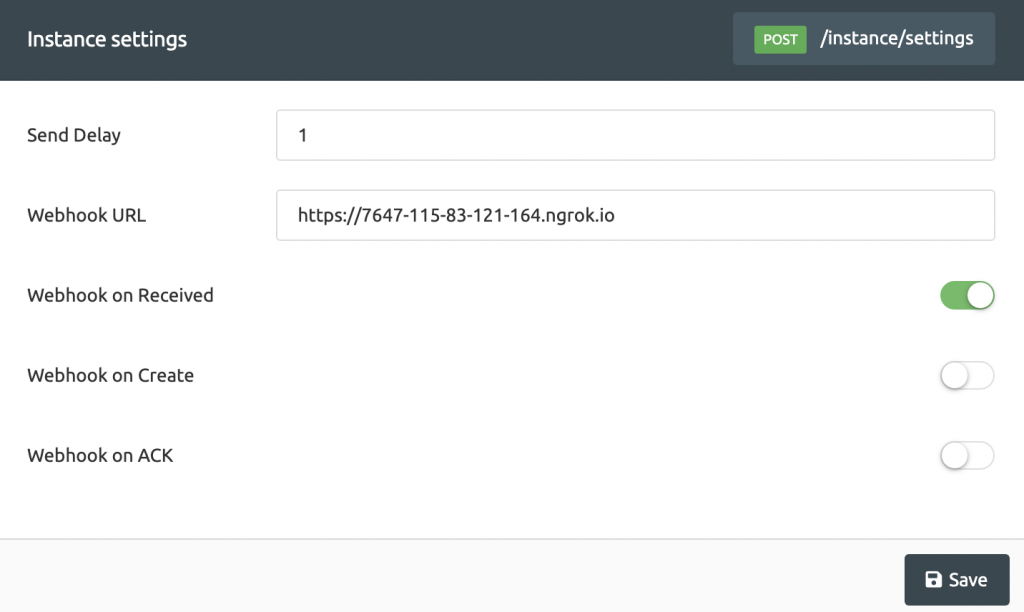
Chatbot Funzioni utilizzate nel codice
invia messaggio
Utilizzato per inviare messaggi di testo WhatsApp
def send_message(self, chatID, text):
data = {"to" : chatID,
"body" : text}
answer = self.send_requests('messages/chat', data)
return answer
- ChatID – ID della chat a cui deve essere inviato il messaggio per lui, ad esempio [email protected] .
- Testo – Testo del messaggio.
volta
Invia l’ora corrente del server.
def time(self, chatID):
t = datetime.datetime.now()
time = t.strftime('%Y-%m-%d %H:%M:%S')
return self.send_message(chatID, time)
- ChatID – ID della chat a cui deve essere inviato il messaggio per lui, ad esempio [email protected] .
invia_immagine
Invia un’immagine al numero di telefono o al gruppo
def send_image(self, chatID):
data = {"to" : chatID,
"image" : "https://file-example.s3-accelerate.amazonaws.com/images/test.jpeg"}
answer = self.send_requests('messages/image', data)
return answer
invia_video
Invia un video al numero di telefono o al gruppo
def send_video(self, chatID):
data = {"to" : chatID,
"video" : "https://file-example.s3-accelerate.amazonaws.com/video/test.mp4"}
answer = self.send_requests('messages/video', data)
return answer
invia_audio
Invia un file audio al numero di telefono o al gruppo
def send_audio(self, chatID):
data = {"to" : chatID,
"audio" : "https://file-example.s3-accelerate.amazonaws.com/audio/2.mp3"}
answer = self.send_requests('messages/audio', data)
return answer
invia_voce
Invia una registrazione audio ppt al numero di telefono o al gruppo
def send_voice(self, chatID):
data = {"to" : chatID,
"audio" : "https://file-example.s3-accelerate.amazonaws.com/voice/oog_example.ogg"}
answer = self.send_requests('messages/voice', data)
return answer
invia_contatto
Invio di un contatto o un elenco di contatti al numero di telefono o al gruppo
def send_contact(self, chatID):
data = {"to" : chatID,
"contact" : "[email protected]"}
answer = self.send_requests('messages/contact', data)
return answer
Elaborazione dei messaggi in arrivo
def Processingـincomingـmessages(self):
if self.dict_messages != []:
message =self.dict_messages
text = message['body'].split()
if not message['fromMe']:
chatID = message['from']
if text[0].lower() == 'hi':
return self.welcome(chatID)
elif text[0].lower() == 'time':
return self.time(chatID)
elif text[0].lower() == 'image':
return self.send_image(chatID)
elif text[0].lower() == 'video':
return self.send_video(chatID)
elif text[0].lower() == 'audio':
return self.send_audio(chatID)
elif text[0].lower() == 'voice':
return self.send_voice(chatID)
elif text[0].lower() == 'contact':
return self.send_contact(chatID)
else:
return self.welcome(chatID, True)
else: return 'NoCommand'
Puoi vedere i passaggi precedenti in questo video:
link utili
- Tutto il codice è disponibile su GitHub .
- invia messaggi API WhatsApp utilizzando Python.
- come impostare WebHook utilizzando la piattaforma Ultramsg
- come ricevere messaggi WhatsApp utilizzando python e webhook.