Introduction
In this tutorial, we will create a page to handle incoming WhatsApp message webhooks using Node.js
Dependencies
for local development purposes, a tunneling service is required. This example uses ngrok, You can download ngrok here: https://ngrok.com/download
Set up your project
install express and body-parser:
npm install express body-parser
A simple example of receiving WhatsApp messages from Ultramsg
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
const PORT = 3000
// Setup a webhook route
app.use(bodyParser.json())
app.post('/ultramsgwebhook', (req, res) => {
console.log(req.body) // print all response
//messageFrom=req.body['data']['from'] // sender number
//messageMsg=req.body['data']['body'] // Message text
res.status(200).end()
})
app.use(bodyParser.json())
app.listen(PORT, () => console.log(`🚀 Server running on port ${PORT}🚀 `))
Start ngrok & Node.js project
Start Ngrok For Windows :
ngrok http 3000
Start Ngrok For Mac :
./ngrok http 3000
Start Nodejs project :
node index.js
Set Webhook URL to your instance in ultramsg
We create a new webhook endpoint: http://your-ngrok.io.com/ultramsgwebhook to handle the request, Now the webhook urls must be placed in the instance in Ultramsg and enable Webhook on Received option, as in the following image:
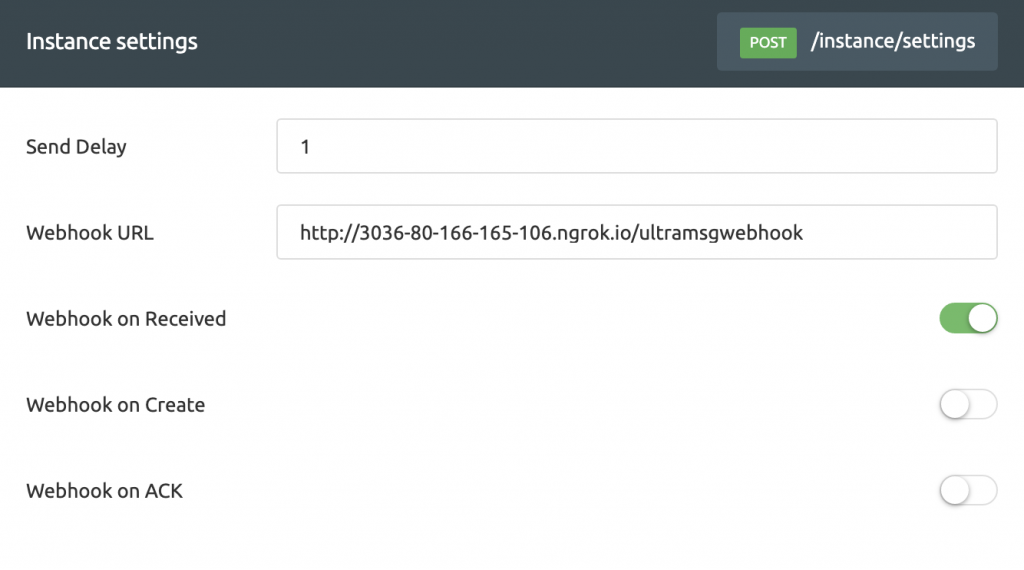
EXAMPLE JSON RESPONSE
{
event_type: 'message_received',
instanceId: '1150',
data: {
id: '[email protected]_3EB0FF54790702367270',
from: '[email protected]',
to: '[email protected]',
ack: '',
type: 'chat',
body: 'Hello, World!',
fromMe: false,
time: 1644957719
}
}
Congratulations. You have received your first WhatsApp message.
Types of webhooks to use with WhatsApp API & Ultramsg
- webhook_message_received : notifications in webhooks when message received .
- webhook_message_create : notifications in webhooks when message create .
- webhook_message_ack : ack (message delivered and message viewed) notifications in webhooks.
- finally, you can see Full Whatsapp API Documentation and FAQ.
- How to Send a Message by WhatsApp API using Node.js easily .
You can see the previous steps in this video :